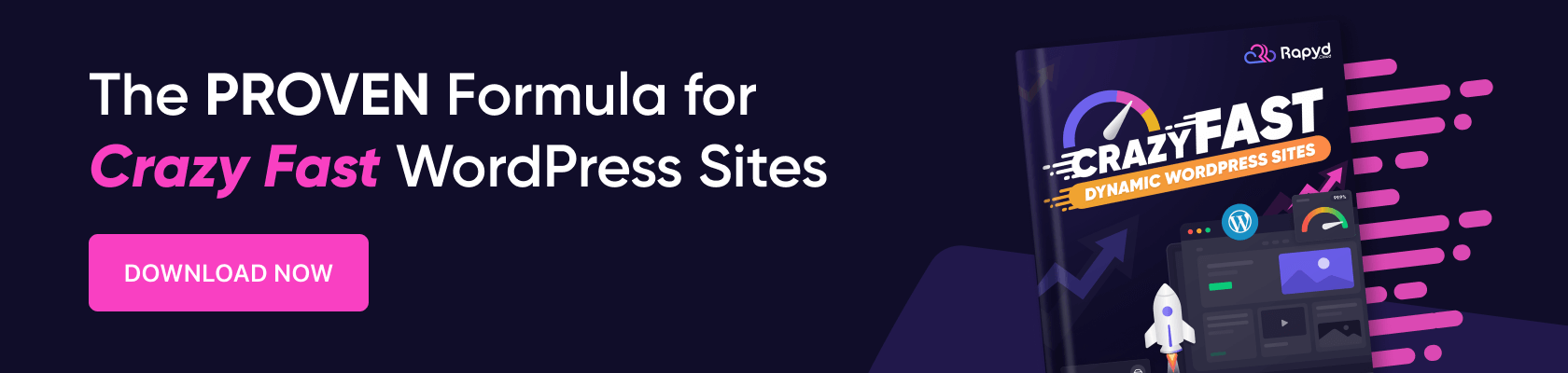
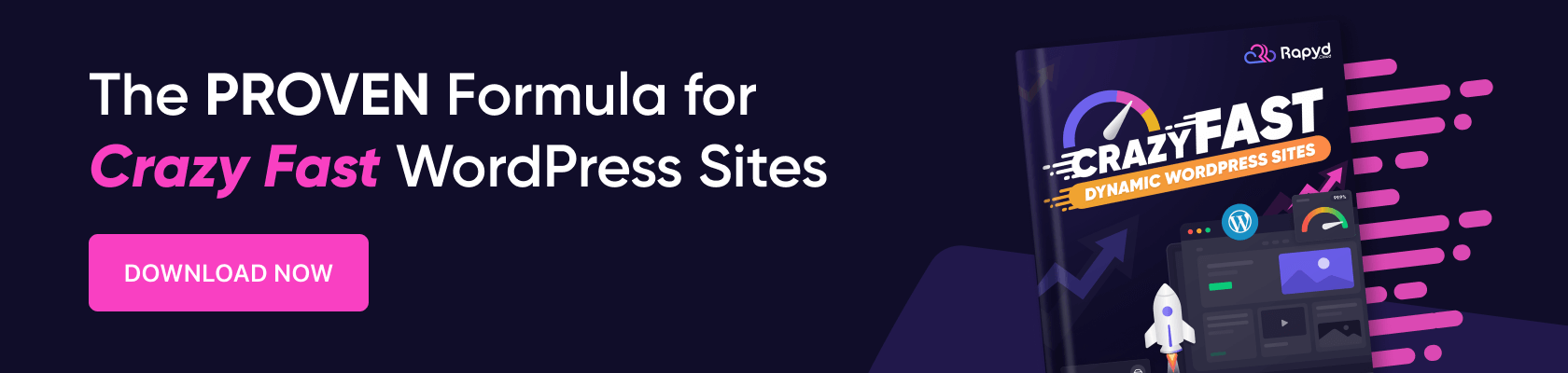
Have you ever wanted to add a new feature to your WordPress site without installing another bulky plugin? This guide will show how a few lines of custom code—these little snippets—can give your website a boost. We’ll cover what these snippets are, how to add them safely, and I’ll share some practical examples you might actually use. Let’s get started!
What Are WordPress Code Snippets?
WordPress code snippets are small building blocks of a website’s functionality. They’re short pieces of code—usually written in PHP, JavaScript, CSS, or HTML—that let you tweak or enhance your site without overcomplicating things. Want a custom login page or a neat automatic redirect after login? Instead of adding a whole plugin just for that one feature, you can simply insert a snippet that does the job.
Typically, WordPress code snippets live in:
- Your theme’s functions.php file
- A child theme (for code changes that survive theme updates)
- A custom plugin
- A dedicated code snippet plugin (like “Code Snippets”)
Which method you choose depends on your comfort level and how frequently you plan to update or change your theme.
Four Ways to Add WordPress Code Snippets
- Editing Theme Files Directly
- You can open your theme’s functions.php (for PHP code) or header.php/footer.php (for HTML/JavaScript).
- Be cautious: A single typo can break your site. Always back up before making direct edits.
- Using a Child Theme
- A child theme inherits styles and functionality from its parent theme but keeps your custom code separate.
- This is one of the safest ways to store WordPress code snippets if you’re comfortable creating a child theme.
- Creating a Custom Plugin
- By placing snippets in a custom plugin, you avoid messing with theme files altogether.
- Perfect if you switch themes often or want a portable set of custom functions.
- Employing a Code Snippets Plugin
- Tools like “Code Snippets” give you an easy interface to add, manage, and deactivate snippets.
- Great for those not comfortable editing PHP directly or who prefer an organized dashboard for their code.
Step-by-Step: Adding Snippets via a Child Theme
- Create (or Install) a Child Theme
- If you’re using a theme named “MyTheme,” you’d create “MyTheme Child” with its own style.css and functions.php.
- This ensures your custom code remains intact even when you update the parent theme.
- Open Your Child Theme’s functions.php
- Go to Appearance > Theme File Editor in WordPress, then pick your child theme from the dropdown.
- Find Theme Functions (functions.php) in the file list.
- Paste Your Code Snippet
- Place your snippet just before the closing ?> tag (if it exists). If there’s no closing tag, simply paste the snippet at the end of the file.
- Save and Test
- After you hit “Update File,” check your site to ensure everything still works.
- If you see an error, revert your changes or restore from a backup.
Step-by-Step: Adding Snippets via the Code Snippets Plugin
- Install and Activate “Code Snippets”
- In the WordPress admin, go to Plugins > Add New, search for “Code Snippets,” then click Install and Activate.
- Access the Snippets Dashboard
- On the left-hand menu, click Snippets. You’ll see a list of active and inactive snippets.
- Add a New Snippet
- Click Add New. Give your snippet a descriptive title.
- In the code editor, paste your snippet. Optionally, add a description or tags.
- Save and Activate
- Click Save Changes and Activate. The snippet now runs on your site.
- If you run into problems, you can easily deactivate it from the same dashboard—no file editing needed.
5 Common WordPress Code Snippets (With Examples)
// Makes the login page logo link to a custom URL
add_filter('login_headerurl','custom_login_link');
function custom_login_link() {
return "https://your-site.com/";
}
- Changes the default WordPress logo link on the login screen to your preferred site.
Separate Homepages for Logged In vs. Logged Out Users
if( is_user_logged_in() ) {
$page = get_page_by_path( 'private-home' );
update_option( 'page_on_front', $page->ID );
update_option( 'show_on_front', 'page' );
} else {
$page = get_page_by_path( 'public-home' );
update_option( 'page_on_front', $page->ID );
update_option( 'show_on_front', 'page' );
}
- Requires you to set the “public-home” or “private-home” slug. Then, it automatically shows a different homepage based on the user’s login status.
Redirect Users to Their Profile Upon Login
function redirect_to_profile( $redirect_to_calculated, $redirect_url_specified, $user ) {
if ( ! $user || is_wp_error( $user ) ) {
return $redirect_to_calculated;
}
if ( empty( $redirect_to_calculated ) ) {
$redirect_to_calculated = admin_url();
}
if ( function_exists( 'bp_core_get_user_domain' ) && ! is_super_admin( $user->ID ) ) {
return "https://your-site.com/user-profile/";
}
return $redirect_to_calculated;
}
add_filter( 'login_redirect', 'redirect_to_profile', 100, 3 );
- Non-admin users go straight to their profile page instead of the WordPress dashboard.
Restrict Admin Area to Admins Only
function restrict_admin_area() {
if ( ! current_user_can( 'manage_options' ) && ! wp_doing_ajax() ) {
wp_safe_redirect( site_url() );
exit;
}
}
add_action( 'admin_init', 'restrict_admin_area', 1 );
- Boots out non-admin users who try to access the WP admin, redirecting them back to the homepage.
Enable Automatic User Registration
function disable_validation( $user_id ) {
global $wpdb;
$wpdb->query( $wpdb->prepare( "UPDATE $wpdb->users SET user_status = 0 WHERE ID = %d", $user_id ) );
}
add_action( 'bp_core_signup_user', 'disable_validation' );
function fix_signup_form_validation_text() {
return false;
}
add_filter( 'bp_registration_needs_activation', 'fix_signup_form_validation_text');
- Lets new users start using their accounts right away, bypassing the usual activation link or manual approval.
Best Practices When Working with WordPress Code Snippets
- Backup First: Always create a backup before you add or modify WordPress code snippets. A single misplaced semicolon can take your site down.
- Understand the Code: Don’t paste random snippets if you’re unsure what they do. Unfamiliar or outdated snippets can introduce security holes.
- Use a Test Environment: If you can, spin up a staging site or local environment to verify your code changes before pushing them live.
- Comment Everything: Leaving notes (// your comment here) ensures you—and anyone else—know the snippet’s purpose months from now.
- Stay Theme-Agnostic: If you use a child theme or custom plugin, you won’t lose your changes when the theme updates.
- Follow Coding Standards: WordPress has coding guidelines for consistency and security. Sticking to them helps avoid weird conflicts.
- Keep It Minimal: The fewer WordPress code snippets you run, the fewer potential conflicts and performance issues. Only add what you actually need.
- Stay Updated: Check occasionally if your code snippet remains compatible with the latest WordPress release. If something breaks, see if you need to adjust or remove it.
- Use Plugins as Needed: If editing code isn’t your style, or you prefer a simpler management interface, code snippet plugins exist for a reason. They make it easier to turn certain snippets on or off quickly.
Conclusion
Well-chosen WordPress code snippets can transform your site’s functionality without burying it under dozens of plugins. Whether you’re customizing your login page, restricting admin access, or automatically directing users to specific pages, these small bits of code can make a big impact. Just remember to back up your site, understand what you’re adding, and ideally test in a safe environment first.
By following best practices and using methods like child themes or snippet management plugins, you’ll keep your customizations manageable, secure, and ready for future WordPress updates. So go ahead—experiment with WordPress code snippets and watch your site evolve in all the right ways. If you’re ever unsure, consulting a pro or using a test site can help you avoid any major hiccups. Happy coding!
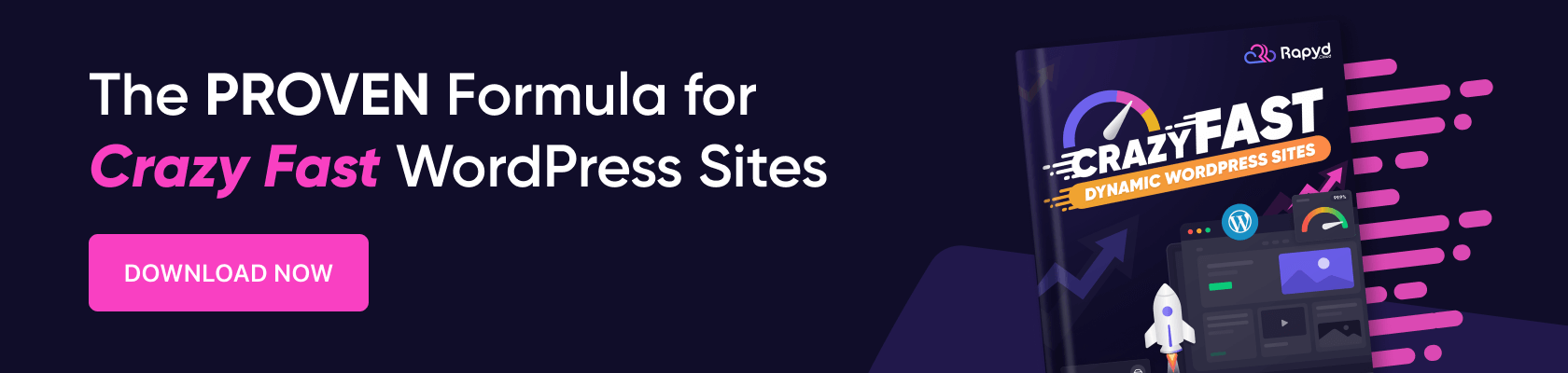